iOS Integration
The Audience SDK is a security-centric tool enabling mobile apps to access location-based advertising.
Overview
The Audience SDK segments mobile app users on-device to offer them relevant location-based ads. Due to the security-centric approach, location and behavioral users' data do not leave their devices. The SDK delivers the segment-relevant ad upon the publisher's app request.
Getting started
Publishers who wish to integrate the Audience SDK into their iOS app can do so by following the steps below.
Step 1: Set Up The Environment
You will need the following prerequisites:
- iOS 14+
- Xcode 14+
- CocoaPods Package Manager
- ClientID and ClientSecret provided by the GroundTruth team
- Your app must have a legitimate interest in collecting users' location data
Step 2: Configure Your Project
- Open your project in Xcode
- Add Background Modes capability in Signing & Capabilities
- Enable Location updates:
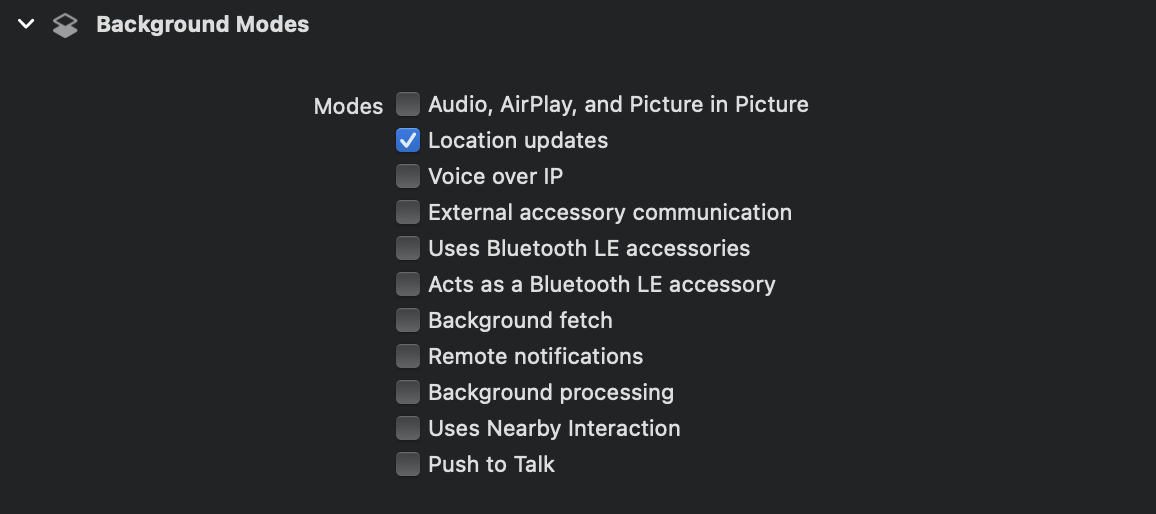
- Right-click the Info.plist file, and choose Open As > Source Code
- Paste the following XML snippet inside
<dict>...</dict>
, replacing<string>
description for each<key>
permission element:
<key>NSLocationAlwaysAndWhenInUseUsageDescription</key>
<string>CUSTOMISE THE PERMISSION REQUEST DESCRIPTION</string>
<key>NSUserTrackingUsageDescription</key>
<string>CUSTOMISE THE PERMISSION REQUEST DESCRIPTION</string>
After the first app launch, a user will see the permission request:
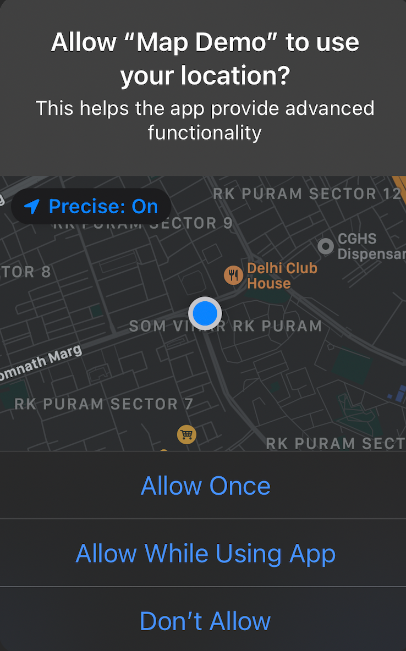
- (Objective-C only) In Build Settings, set Always Embed Swift Standard Libraries to Yes:

Step 3: Install The SDK
- Create a Podfile in the project directory:
pod init
- Edit the Podfile as follows:
platform :ios, '14.0'
use_frameworks!
target "TYPE_YOUR_APP_NAME" do
pod 'GroundTruthAudienceSDK'
end
- Install the pod:
pod install
Step 4: Initialize the SDK
- Open your app's main entry point
- Paste the code snippet with the ClientID and ClientSecret credentials:
import GroundTruthAudienceSDK
let clientId = “TYPE_YOUR_CLIENT_ID”
let clientSecret = “TYPE_CLIENT_SECRET”
let thirdPartyId = ThirdPartyId.init(
source: Bundle.main.bundleIdentifier,
version: Bundle.main.infoDictionary["CFBundleShortVersionString"],
id: UUID.init().uuidString
)
let configuration = AudienceClientConfiguration(
clientId: clientId,
clientSecret: clientSecret,
thirdPartyId: thirdPartyId
)
@import GroundTruthAudienceSDK;
NSString *clientId = @"TYPE_YOUR_CLIENT_ID";
NSString *clientSecret = @"TYPE_CLIENT_SECRET";
ThirdPartyId *thirdPartyId = [[ThirdPartyId alloc]
initWithSource:NSBundle.mainBundle.bundleIdentifier
version:NSBundle.mainBundle.infoDictionary["CFBundleShortVersionString"]
d:NSUUID.UUID.UUIDString
];
AudienceClientConfiguration *configuration =
[[AudienceClientConfiguration alloc]
initWithClientId:clientId
clientSecret:clientSecret
thirdPartyId:thirdPartyId
];
- Paste the following code block with your:
- Ad-framework processing the audience list
- Error handling
AudienceSDK.shared.start(withConfiguration: configuration){
startupResult in
switch(startupResult) {
case .success(()):
// Insert an ad framework that processes the audience list updates
break
case .failure(let error):
// Insert error handling
break
}
}
static NSString * const getAudienceSdkQueueName()
{
static dispatch_once_t onceToken;
__block NSString *_audienceSdkQueueName;
dispatch_once(&onceToken, ^
{
_audienceSdkQueueName = [
NSString stringWithFormat:@"%@.audienceSdk",
NSBundle.mainBundle.bundleIdentifier
];
});
return _audienceSdkQueueName;
}
static dispatch_queue_t getAudienceSdkQueue()
{
static dispatch_once_t onceToken;
static dispatch_queue_t _audienceSdkQueue;
dispatch_once(&onceToken, ^
{
_audienceSdkQueue = dispatch_queue_create(
[getAudienceSdkQueueName() UTF8String], NULL
);
});
return _audienceSdkQueue;
}
[[AudienceSDK shared] startWithConfiguration:audienceSdkConfig
returnOnQueue:getAudienceSdkQueue()
onError:^(NSError * error)
{
// Insert error handling
return;
}
onSuccess:^
{
[[AudienceSDK shared] getAudiencesWithReturnOnQueue:getAudienceSdkQueue()
onError:^(NSError * error)
{
// Insert error handling
return;
}
onSuccess:^(GTAudienceResultObjc * result)
{
self.audiences = result;
// Insert an ad framework that processes the audience list updates
return;
}
];
return;
}
];
The Audience SDK runs in the background indefinitely. If you wish to disable it, add and call the following method:
AudienceSDK.stop()
[[AudienceSDK shared] stop];
Test The Integration
Get in touch with the GroundTruth team to test the SDK integration.
SDK FAQs & troubleshooting
- What analytics does the SDK share with GroundTruth?
- What is the update frequency?
- Need help finding an answer to your question?
What analytics does the SDK share with GroundTruth?
The SDK shares the following information with the GroundTruth servers:
- Events' logs while the publisher's app is running
- Permissions required for location and motion use
- Device metadata
The analytics have the following structure:
{
"events" : [
{
"Type": "EventType (from the list above)",
"ts": "An integer",
"data": "An event object serialized binary representation"
},
{
"…"
},
"… more events …"
]
}
What is the update frequency?
The analytics are shared with the GroundTruth every 30 minutes. The SDK will repeat the request every three minutes if no network is available.
Need help finding an answer to your question?
If you have encountered any issues, contact the GroundTruth team for assistance.
Updated 11 months ago