iOS SDK Integration
This is the integration document for the GroundTruth Location SDK.
The Location SDK collects location signals from a device on which the publisher app is installed.
Our platform uses the location data for audience creation, ads serving, offline visit attribution and brand insights generation.
Latest Release (Version 5.3.3)
Change Logs
- Fix for performance issues
Integration
Requirements
- iOS 10 or greater
- The App must have legitimate interest in collecting users location data
- This version currently supports xCode 13
Step 1 - Obtain GT Access Key & Password
Your account manager will provide you with a unique access_key
& password
.
If you have not received them, please contact your GT account manager.
Step 2 - Install CocoaPods
Create a pod file, with the following command in the terminal:
pod init
Edit your Podfile as following:
platform :ios, '14.0'
use_frameworks!
target "YOUR_APP_NAME" do
pod 'GroundTruthLocationSDK'
end
Install pods by running the following command in the terminal:
pod install
Step 3 - Enable Background Modes - Background Fetch
Under background mode, enable Location Updates and Background fetch . This enables the SDK to track the location when the app is running in the background.
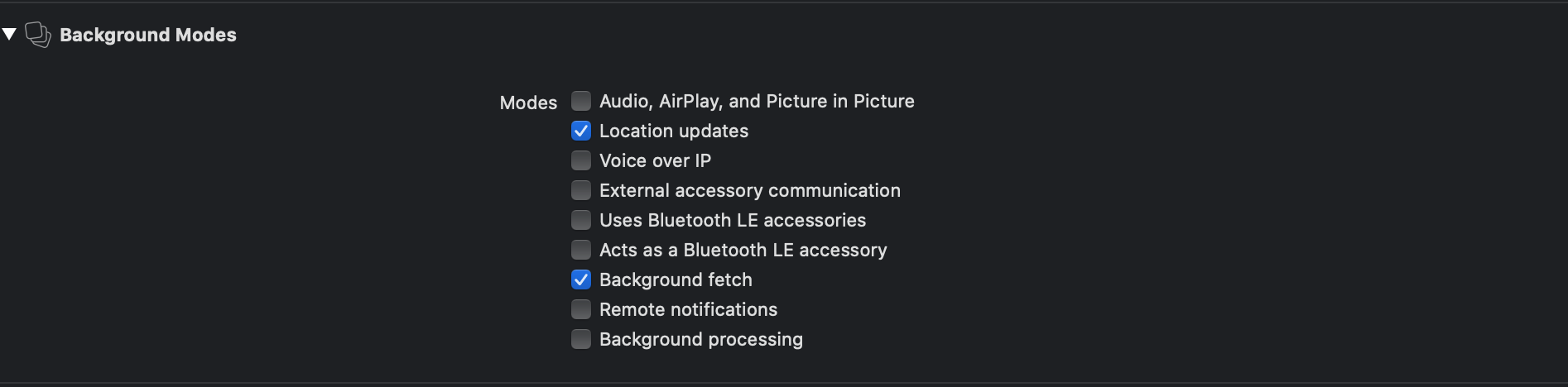
If you haven't done so already - customize the location sharing opt-in message your users will see during the application’s first launch. Add a convincing description as to why the user should grant his permission for sharing his location (preferably background location).
Place your customized message to the NSLocationAlwaysUsageDescription, NSLocationWhenInUseUsageDescription, NSLocationAlwaysAndWhenInUseUsageDescription and NSUserTrackingUsageDescription keys into the Info.plist.

Step 4 (Objective-C only)
In Project Settings, General tab - add Cocoapods / Location SDK framework into Embedded Binaries.

Step 5 (Objective-C only)
In project settings, Build Settings tab and set Always Embed Swift Standard Libraries to "YES"

Step 6 - Get the desired location permission
The SDK will not power up unless the user has opted-in to share his location.
If the user has selected “Allow While Using App”, to make the App keep on fetching location updates in the background, the user has to go into the Settings, search the App and enable Always for location services.
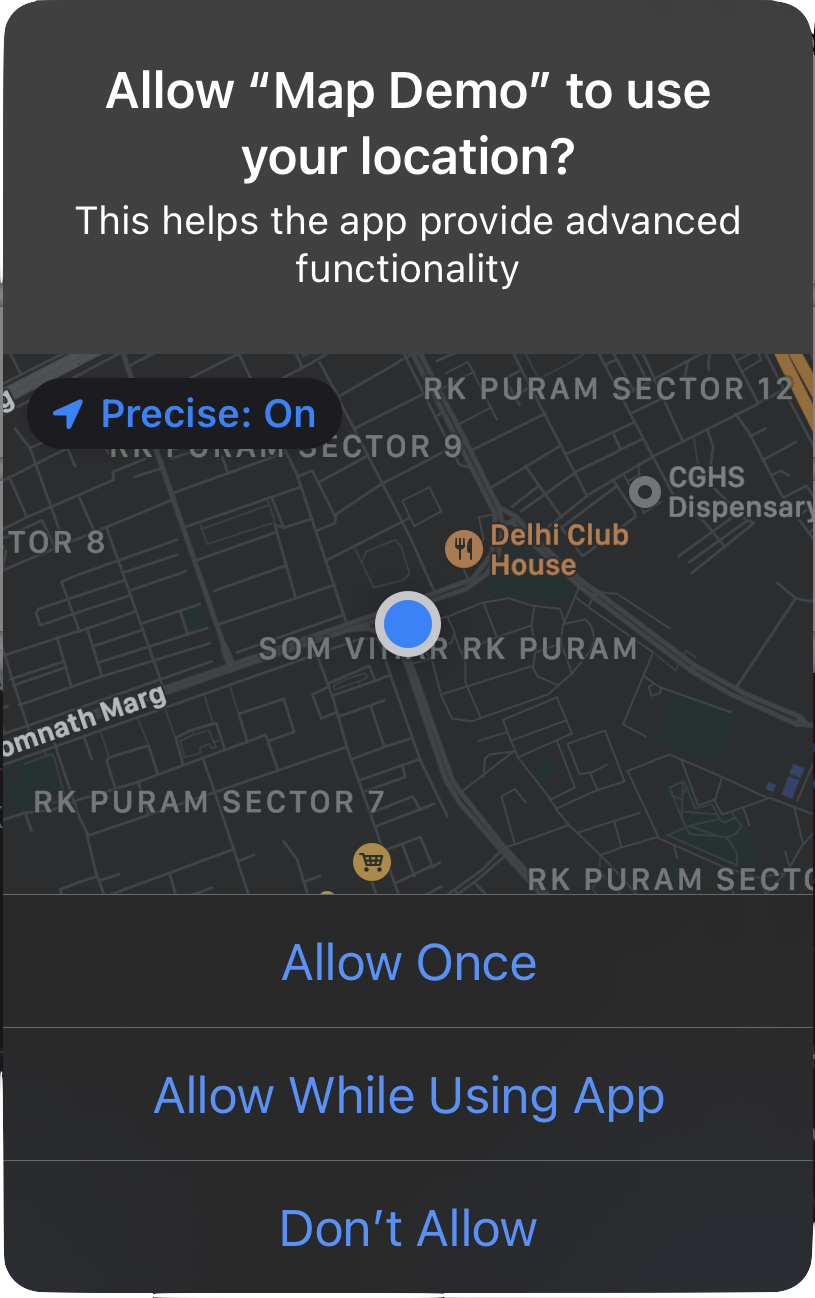
Or if the user has not selected the “Allow While Using App”, then iOS will automatically prompt the user for allowing the location updates in the background.
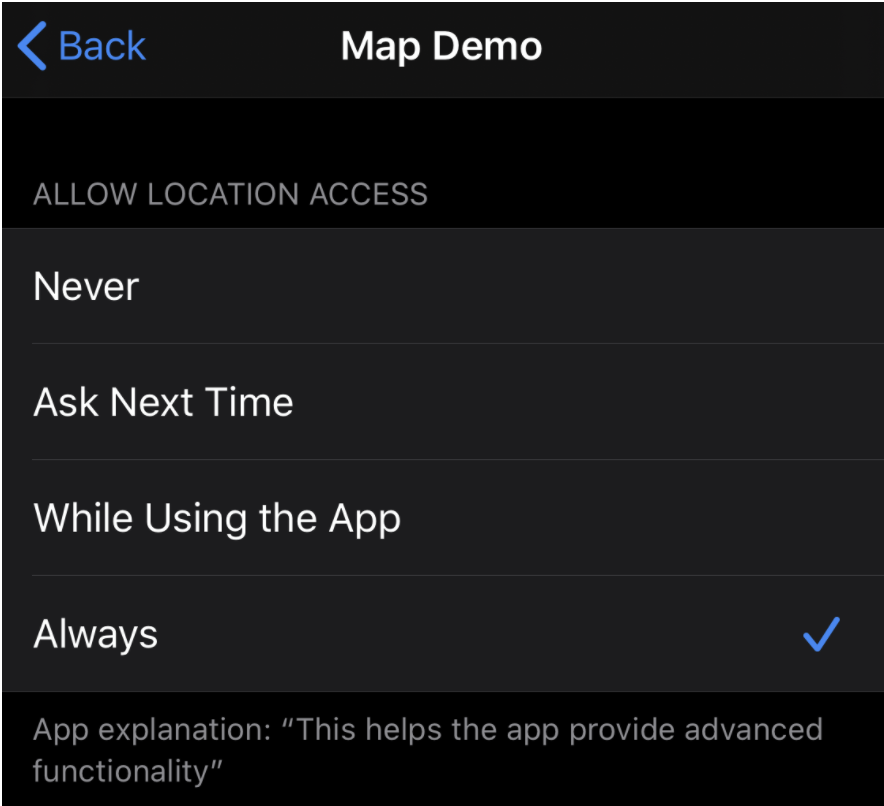
Step 7 - Initialize the SDK
Add the SDK initialization code in the AppDelegate under didFinishLaunchingWithOptions and replace the access_key and password with the one provided by GroundTruth.
Note: userGender and userBirthday are optional.
Set gdpr - false whenever a user is not restricted by GDPR, and true if the user is restricted by GDPR.
If the user is located in EU/EEA then this field must be set to true.
consent is the IAB consent string for GDPR which is a daisybit encoded in base64.
XADLocationSDK.configSDK(accessKey:"<access_key>",
password: "<password>",
userGender: .male)
[XADLocationSDK configSDK: @"<access_key>"
password: @"<password>"
userBirthday: nil
userGender: GenderMale
gdpr: false
consent: nil
ccpaPrivacyString:nil];
Step 8 - User opt-in
Ask the user to opt-in for location sharing.
By default, we will be requesting AlwaysOn, but you can use the overloaded method to allow you to switch to WhileInUse location access.
Use this to present Location Activity permission dialog to the user:
XADLocationSDK.requestAuthorization()
//OR
XADLocationSDK.requestAuthorization(authorizationType: .whileInUse)
[XADLocationSDK requestAuthorization];
//OR
[XADLocationSDK requestAuthorizationWithAuthorizationType: LocationAuthorizationTypeWhileInUse]
Ask the user to opt-in for App Tracking Authorization using the requestPermission() method.
By default, we will be requesting Authorized, but the user can choose as per preference.
@available(iOS 14, *)
func requestPermission() {
// Ask for App Tracking permission
ATTrackingManager.requestTrackingAuthorization { status in
// Ask for Always location permission
XADLocationSDK.requestAuthorization(authorizationType: .always)
}
}
Step 9 - Enable background mode
Add the following lines of code in AppDelegate to help make sure that your app (and XADLocationSDK) continues to run in the background correctly.
func application(application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool
{
// CONFIGURE BACKGROUND FETCH FOR BACKGROUND SDK USAGE
application.setMinimumBackgroundFetchInterval(
UIApplicationBackgroundFetchIntervalMinimum)
return true
}
func application(application: UIApplication,
performFetchWithCompletionHandler completionHandler: (UIBackgroundFetchResult) -> Void)
{
completionHandler(.newData)
- (BOOL)application:(UIApplication *)application
didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
// CONFIGURE BACKGROUND FETCH FOR BACKGROUND SDK USAGE
[[UIApplication sharedApplication] setMinimumBackgroundFetchInterval:UIApplicationBackgroundFetchIntervalMinimum];
return YES;
}
- (void)application:(UIApplication *)application
performFetchWithCompletionHandler:(void (^)(UIBackgroundFetchResult result))completionHandler
{
completionHandler(UIBackgroundFetchResultNewData);
}
Additional Options
Enable Logs
This option can be used to check the Location SDK status logs. Here's an example of how to enable logs:
XADLocationSDK.enableLogs()
[XADLocationSDK enableLogs];
Full Example of Integration
import XADLocationSDK
func application(application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool
{
let formatter = DateFormatter()
formatter.dateFormat = "MM/dd/yyyy"
let birthday = formatter.date(from: "<MM/dd/yyyy>")
XADLocationSDK.configSDK(accessKey:"<access_key>",
password: "<password>",
userBirthday: birthday,
userGender: .male,
gdpr: false,
consent: nil,
ccpaPrivacyString: nil)
XADLocationSDK.requestAuthorization()
// CONFIGURE BACKGROUND FETCH FOR BACKGROUND SDK USAGE
application.setMinimumBackgroundFetchInterval(
UIApplicationBackgroundFetchIntervalMinimum)
return true
}
func application(application: UIApplication,
performFetchWithCompletionHandler completionHandler: (UIBackgroundFetchResult) -> Void)
{
completionHandler(.newData)
}
#import <XADLocationSDK/XADLocationSDK-Swift.h>
- (BOOL)application:(UIApplication *)application
didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
NSDateFormatter *formatter = [[NSDateFormatter alloc] init];
[formatter setDateFormat:@"MM/dd/yyyy"];
NSDate *birthday = [formatter dateFromString:@"<MM/dd/yyyy>"];
[XADLocationSDK configSDKWithAccessKey: @"<access_key>"
password: @"<password>"
userBirthday: birthday
userGender: GenderMale
gdpr: false
consent: nil
ccpaPrivacyString: nil];
[XADLocationSDK requestAuthorization];
// CONFIGURE BACKGROUND FETCH FOR BACKGROUND SDK USAGE
[[UIApplication sharedApplication] setMinimumBackgroundFetchInterval:UIApplicationBackgroundFetchIntervalMinimum];
return YES;
}
- (void)application:(UIApplication *)application
performFetchWithCompletionHandler:(void (^)(UIBackgroundFetchResult result))completionHandler
{
completionHandler(UIBackgroundFetchResultNewData);
}
Updated over 2 years ago